MAKAUT BTech CSE/IT Data Structure concepts
Answer:
Pointers may be used in a code in two different ways.
1. Dynamic Memory Allocation:
For efficient memory management, we can allocate memory to store data at run-time. If we use an array, the size of the array is fixed at compilation time. So, we always try to create a significantly large array to store all possible data. This increases the wastage of memory as all of the memory, allocated for the array, may not be used at run-time (i.e., while execution). Using dynamic memory allocation, we can create (malloc() and calloc() functions) or remove (free() function) space for variables as and when needed.
Creation of a dynamic array:
// if n is a user input (integer), the following statement creates an array of length n.
int* array_ptr = (int *)malloc(n * sizeof(int));
// To delete the entire array
free(array_ptr
); //deallocates memory
2. Parameter passing between functions (so-called Call-By-Reference):
When a function is called, the caller function can send the address of some variable to the called function. The called function must use a pointer to hold that address. Thus, the called function can access and update the memory location of the variable whose address has been passed to it. All the changes made by the called function are stored in the variable of the called function. So, when the called function returns (and is removed from memory), the changes it has made will persist.
Explanation
The following example has two functions to swap the values passed from the main function (caller function, in this case).
In swap(), the values of x and y from main() ara copied to the variables named a and b in swap(). All these variables are auto (automatic) variables and local in scope. So, although the values of a and b have been swapped within the swap(), the values of x and y in main() remain the same (see sample output).
In the case of swap2(), the function is having two pointer variables (p1 and p2). Though these two pointers are also local and auto in nature, but these pointers are set with the addresses of x and y, respectively, from the caller function (main() in this case). So, the swap operation performed through p1 and p2 is actually performed in the memory locations of x and y. Thus, the result of swap2() will persist even when the function ends.
Example:
The following code snippet shows how to use pointers as a formal parameter.
#include<stdio.h>
void swap(int a, int b) { // Call by value
int temp;
temp = a;
a = b;
b = temp;
return;
}
void swap2(int *p1, int *p2){ // Call by reference
int temp;
temp = *p1;
*p1 = *p2;
*p2 = temp;
return;
}
int main(){
int x = 5, y = 10;
printf("\n Before swap() : x = %d, y = %d", x, y);
swap(x, y);
printf("\n After swap() : x = %d, y = %d", x, y);
swap2(&x, &y);
printf("\n After swap2() : x = %d, y = %d", x, y);
return 0;
}
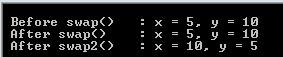
Related Question:
- Can we reuse the same pointer variable to point to some other array after deleting the previously allocated dynamic array?
- What happens if we do not use the free() after using the dynamically allocated array?
- Differentiate between call by value and call by reference in C language.
- Is call by value and call by reference almost the same in C language?
- Why the changes made in “call by value” do not persist?
- How much memory overhead is there in “call by reference” over “call by value” C language??
gSJqyOFIsLerYTx